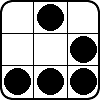 |
Aether3D
Game Engine
|
45 virtual void Draw(
const Texture* texture,
int x,
int y,
const Vec4& color ) = 0;
57 virtual void Draw(
const Texture* texture,
int x,
int y,
int width,
int height,
const Vec4& color ) = 0;
69 virtual void Draw(
const char* text,
const char* fontName,
float scale,
int x,
int y,
const Vec4& color ) = 0;
85 virtual void DrawLines(
const Vec3* lineArray,
int arraySize,
const Vec4& color ) = 0;
94 virtual double GetTime()
const = 0;
97 virtual int Height()
const = 0;
107 virtual Error::Enum
LoadFont(
const char* fontName,
const char* fontBitmapPath,
const char* fontMetadataPath ) = 0;
118 virtual Error::Enum
OpenWindow(
int width,
int height,
bool fullScreen,
int antialiasSamples,
bool hasWindowAndContext ) = 0;
124 virtual void Resize(
int width,
int height ) = 0;
133 virtual void SetBloom(
bool enable,
float factor,
int blurIterations ) = 0;
142 virtual void SetClearColor(
float red,
float green,
float blue ) = 0;
151 virtual void SetSSAO(
bool enable,
float radius,
float power ) = 0;
160 virtual int Width()
const = 0;
virtual void SetBloom(bool enable, float factor, int blurIterations)=0
Stores scene in a scene graph.
Definition: Scene.hpp:28
virtual void Draw(const Texture *texture, int x, int y, const Vec4 &color)=0
virtual bool HasFocus() const =0
virtual void BeginQuery()=0
virtual void DrawStatistics()=0
virtual void FinishFrame()=0
2D or Cube Map texture.
Definition: Texture.hpp:7
virtual void SetClearColor(float red, float green, float blue)=0
virtual void Resize(int width, int height)=0
virtual void SwapBuffers()=0
virtual double GetTime() const =0
virtual void AddShaderSearchPath(const char *path) const =0
Adds a path where Material will search for shaders. Paths are searched in order they are added and th...
3-component vector.
Definition: Vec3.hpp:22
virtual void DrawLines(const Vec3 *lineArray, int arraySize, const Vec4 &color)=0
virtual Error::Enum OpenWindow(int width, int height, bool fullScreen, int antialiasSamples, bool hasWindowAndContext)=0
virtual void SetSSAO(bool enable, float radius, float power)=0
4-component vector.
Definition: Vec3.hpp:380
Renders models and UI.
Definition: Renderer.hpp:14
virtual int Height() const =0
virtual void ClearScreen() const =0
static const unsigned apiVersion
Definition: Renderer.hpp:18
virtual double EndQuery()=0
virtual void SetWindowTitle(const char *title) const =0
virtual Error::Enum LoadFont(const char *fontName, const char *fontBitmapPath, const char *fontMetadataPath)=0
virtual int Width() const =0