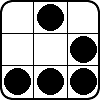 |
Aether3D
Game Engine
|
4 #ifndef INCLUDE_STRING_H
6 # define INCLUDE_STRING_H
8 #ifndef INCLUDE_VECTOR_H
10 # define INCLUDE_VECTOR_H
12 #ifndef SCENEGRAPHNODE_H
13 # include "SceneGraphNode.hpp"
33 virtual std::vector< std::string >
GetMeshNames()
const = 0;
41 virtual std::vector< std::string >
GetMeshJointNames(
const std::string& meshName )
const = 0;
44 virtual const std::string&
GetPath()
const = 0;
52 virtual void ApplyAnimation(
int firstKeyFrame,
int lastKeyFrame ) = 0;
virtual std::vector< std::string > GetMeshJointNames(const std::string &meshName) const =0
Stores material properties for Mesh. Their names/values are directly mapped into shader uniforms.
Definition: Material.hpp:19
virtual void EnableWireframe(bool enable)=0
virtual void ApplyAnimation(int firstKeyFrame, int lastKeyFrame)=0
3-component vector.
Definition: Vec3.hpp:22
virtual void GetAABB(Vec3 &outMin, Vec3 &outMax) const =0
virtual std::vector< std::string > GetMeshNames() const =0
Stores an orientation.
Definition: Quaternion.hpp:17
virtual const std::string & GetPath() const =0
Contains meshes loaded from Aether3D's own .ae3d file format.
Definition: Model.hpp:21
virtual Material * GetMeshMaterial(const char *meshName)=0
virtual void SetMaterialForMeshSubstring(Material *material, const std::string &meshNameSubstring)=0
Scene Graph Node. Models, Lights and Cameras are derived from this class.
Definition: SceneGraphNode.hpp:28
virtual void SetMaterialForMesh(Material *material, const std::string &meshName)=0