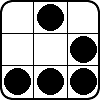 |
Aether3D
Game Engine
|
8 #if defined( _MSC_VER ) || defined( COMPILER_INTEL )
9 # define BEGIN_ALIGNED( n, a ) __declspec( align( a ) ) n
10 # define END_ALIGNED( n, a )
11 #elif defined( __GNUC__ ) || defined( __clang__ )
12 # define BEGIN_ALIGNED( n, a ) n
13 # define END_ALIGNED( n, a ) __attribute__( (aligned( a ) ) )
44 static void InverseTranspose(
const float m[ 16 ],
float* out );
60 static void TransformPoint(
const Vec4& vec,
const Matrix44& mat,
Vec4* out );
69 static void TransformPoint(
const Vec3& vec,
const Matrix44& mat,
Vec3* out );
78 static void TransformDirection(
const Vec3& dir,
const Matrix44& mat,
Vec3* out );
89 MakeRotationXYZ( xDeg, yDeg, zDeg );
100 explicit Matrix44(
const float* data );
117 void Scale(
float x,
float y,
float z );
120 void InitFrom(
const float* data );
132 void MakeLookAt(
const Vec3& eye,
const Vec3& center,
const Vec3& up );
143 void MakeProjection(
float fovDegrees,
float aspect,
float nearDepth,
float farDepth );
155 void MakeProjection(
float left,
float right,
float bottom,
float top,
float nearDepth,
float farDepth );
164 void MakeRotationXYZ(
float xDeg,
float yDeg,
float zDeg );
170 void Transpose(
Matrix44& out )
const;
173 void Translate(
const Vec3& v );
Matrix44()
Constructor. Inits to identity.
Definition: Matrix.hpp:81
3-component vector.
Definition: Vec3.hpp:22
4-component vector.
Definition: Vec3.hpp:380
static const Matrix44 bias
Converts depth coordinates from [-1,1] to [0,1]. This is needed to sample the shadow map texture.
Definition: Matrix.hpp:27
static const Matrix44 identity
Identity matrix.
Definition: Matrix.hpp:24
float m[16]
Member data, row-major.
Definition: Matrix.hpp:176
Matrix44 & operator=(const Matrix44 &other)
Definition: Matrix.hpp:106
Row-major 4x4 Matrix.
Definition: Matrix.hpp:20
Matrix44(float xDeg, float yDeg, float zDeg)
Constructor that makes an XYZ rotation matrix.
Definition: Matrix.hpp:87